This nimib document provides a brief description and example for all blocks in nimib.
Table of Contents:
- nbText
- nbCode
- nbCodeSkip
- nbCodeInBlock
- nbCapture
- nbTextWithCode
- nbImage
- nbVideo
- nbAudio
- nbFile
- nbRawHtml
- nbShow
- nbClearOutput
- nimibCode
nbText
nbText
is a block that displays text. It is the one you will use the most.
You can use markdown syntax to format your text.
The markdown syntax is explained in the Markdown Cheatsheet.
nbText: """
#### Markdown Example
My **text** is *formatted* with the
[Markdown](./cheatsheet.html) syntax.
"""
Markdown Example
My text is formatted with the Markdown syntax.
nbCode
You can insert your Nim code in your text using the nbCode
block.
It displays your code with highlighting, compiles it, runs it and captures any output that is echo
ed.
nbCode:
echo "Hello World!"
echo "Hello World!"
Hello World!
nbCodeSkip
Similar to nbCode
, nbCodeSkip
is a block that displays
highlighted code but does not run it.
It is useful to show erroneous code or code that takes too long to run.
⚠️
nbCodeSkip
does not guarantee that the code you show will compile nor run.
Examples:
nbCodeSkip:
while true:
echo "Notice how there is no output?"
echo "The code is not compiled or executed so no output is generated!"
nbCodeSkip:
exit() # even this won't execute!
while true:
echo "Notice how there is no output?"
echo "The code is not compiled or executed so no output is generated!"
exit() # even this won't execute!
nbCodeInBlock
Sometimes, you want to show similar code snippets with the same variable names and you need to declare twice the same variables.
You can do so with nbCodeInBlock
which nests your snippet inside a block.
nbCode:
var x = true # x is shared between all code blocks
nbCodeInBlock:
var y = 2 # y is local to this block
echo x, ' ', y # The x variable comes from above
nbCodeInBlock:
var y = 3 # We have to redefine the variable y in this new CodeInBlock
echo y
when false:
echo y # This would fail as defined in another scope
var x = true # This would fail in nbCode, since it is a redefinition
var x = true # x is shared between all code blocks
var y = 2 # y is local to this block
echo x, ' ', y # The x variable comes from above
true 2
var y = 3 # We have to redefine the variable y in this new CodeInBlock
echo y
3
nbCapture
nbCapture
is a block that only shows the captured output of a code block.
nbCapture:
echo "Captured!"
Captured!
nbTextWithCode
nbText
only stores the string it is given, but it doesn't store the code passed to nbText
. For example, nbText: fmt"{1+1}"
only stores the string "2"
but not the code fmt"{1+1}"
that produced that string. nbTextWithCode
works like nbText
but it also stores the code in the created block. It can be accessed with nb.blk.code
right after the nbTextWithCode
call. See the end of
numerical for an example.
nbImage
nbImage
enables to display your favorite pictures.
Most formats (.jpg, .png) are accepted. The caption is optional!
nbImage(url="images/todd-cravens-nimib-unsplash.jpg", caption="Blue Whale (photograph by Todd Cravens)")
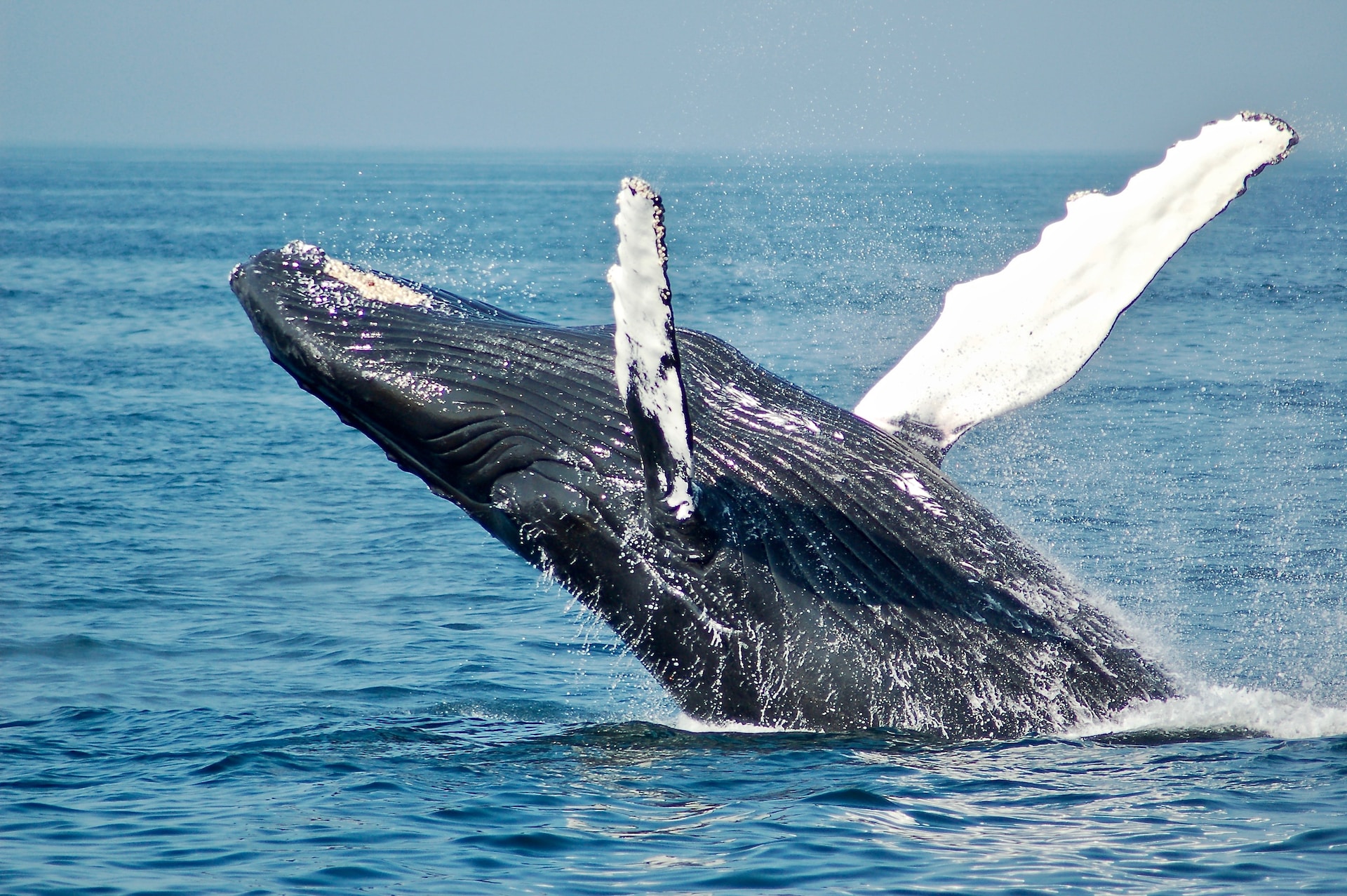
nbVideo
nbVideo
allows you to display videos within your nimib document. You may choose if the
video autoplays, loops, and/or is muted.
Pictured below is Bad Apple!! If you aren't sure what it is, or are curious about its origins, here's an explainer.
The typ
parameter specifies the video's MIME type, and is optional!
nbVideo(url="media/bad_apple!!.mp4", typ="video/mp4")
nbAudio
nbAudio
enables you to play audio in nimib. You may choose if the audio autoplays,
loops, and/or is muted.
The typ
parameter is similar to nbVideo
's.
nbAudio(url="media/bad_apple!!.webm", loop=true)
nbFile
nbFile
can save the contents of block into a file or display the contents of a file.
To save to a file it takes two arguments: the name of the file and the content of the file. The content can be a string or a code block.
nbFile("exampleCode.nim"):
echo "This code will be saved in the exampleCode.nim file."
exampleCode.nim
echo "This code will be saved in the exampleCode.nim file."
To display a file, it takes one argument: the file's path.
nbFile("../LICENSE")
../LICENSE
MIT License
Copyright (c) 2020 pietroppeter
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
nbRawHtml
Certain things are not doable with pure Markdown. You can use raw HTML directly with the nbRawHtml
block.
For example, you have to use HTML style attribute and inject CSS styling in a HTML tag to center your titles (that is a Markdown limitation).
Here is the source code and the centered title:
nbRawHtml: """<h4 style="text-align: center">Centered title</h4>"""
Centered title
nbShow
Nimib allows to pretty-print objects by rendering them to HTML. If the object has an associated toHtml()
procedure, it can be rendered with nbShow
.
import datamancer
let s1: seq[int] = @[22, 54, 34]
let s2: seq[float] = @[1.87, 1.75, 1.78]
let s3: seq[string] = @["Mike", "Laura", "Sue"]
let df = toDf({ "Age" : s1,
"Height" : s2,
"Name" : s3 })
nbShow(df)
Index | Age int | Height float | Name string |
---|---|---|---|
0 | 22 | 1.87 | Mike |
1 | 54 | 1.75 | Laura |
2 | 34 | 1.78 | Sue |
nbClearOutput
Clears the output of the preceding code block if you do not want to show it. This block is useful if you produce too long output or you do not want to show it.
It comes in handy when you want to plot with ggplotnim
without the additional output that the library produces.
nbCode:
var i = 0
while i < 1000:
echo i
inc i
nbClearOutput
var i = 0
while i < 1000:
echo i
inc i
No output !!
nimibCode
nimibCode
(do not be confused with nbCode
) is a special block designed for those that want to spread their love of nimib.
It displays the code of a nimib document (even nbCode blocks), compiles and runs the block, and displays the potential output.
By clicking on the "Show source" button, you can see the multiple usages of this block in this document.
Happy coding!